January 6, 2024, 11:46 am
If you are like me (and you know you are), I like to regularly sync my iPhone to my MacBook Pro. This is mainly just for tracking what music I listen to, as I do not do backups to my local computer any more.
Alas, there is a fly in the ointment. Lately I have been plugging my iPhone into my MBP, and then from Finder I click on the iPhone on the left and click the Sync button, and along the bottom it stays at “Syncing (Step 2 of 4) — Preparing to sync” and never moves. Here is an example of what it looks like: (You’ll forgive me if I redact some of the more sensitive bits here.)
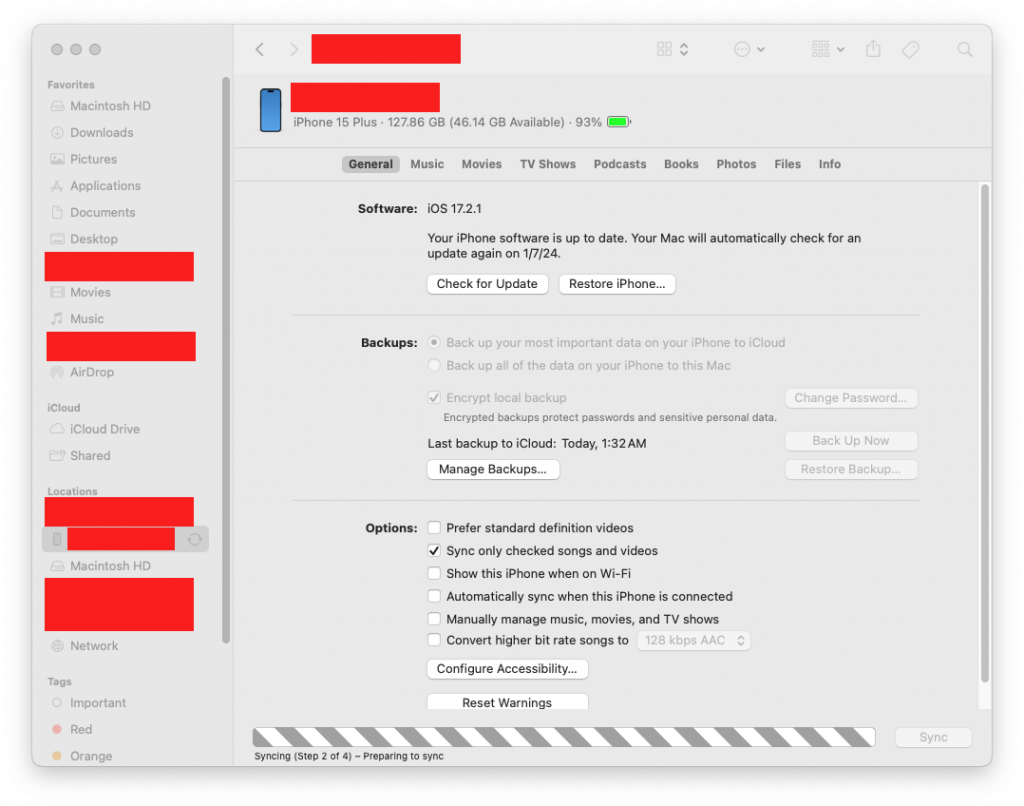
By the way, this is an Apple Silicon MBP and it is on the latest Sonoma.
I have a solution that seems to work for me, but keep in mind that your mileage may vary, especially since the workaround that I am talking about involves terminating a system process.
So as it turns out, there is a system process running called “MDCrashReportTool” that is somehow interfering with this process. The way that I have been able to get this to complete is to launch the Activity Monitor, then from the CPU tab search for “crash”, and your window will then look something like this:

Double click on the MDCrashReportTool entry, and from the dialog that pops up click Quit, and then click Force Quit. Once you do this, your sync should go through without incident, even if it was hung on step 2 of 4 when you kill the MDCrashReportTool process.
You will have to do this every time you reboot the computer, as the MDCrashReportTool seems to reappear again for me.
December 27, 2023, 12:42 pm
There is no worse feeling in the world than when you load up an old Objective-C project that you have to make some changes on, and all of a sudden there are no schemes present for the project when you pull it up in Xcode. As such, there is no way to run the project to see if your changes are good or not.
I did find a solution to this issue. Apparently you need to have Xcode rebuild some of your user data to get the schemes back again.
If this is happening to you when you load your project or workspace into Xcode, do the following steps:
- With the project/workspace open in Xcode, right click on the project item all the way at the top of the Project navigator, and select “Show in Finder”.
- Quit Xcode.
- From the Finder window that just opened, right click on the .xcodeproj file for your project and select “Show Package Contents”.
- Double click on the xcuserdata folder.
- Right click on the folder that begins with your user name and ends with .xcuserdatad and select “Move to Trash”.
- If you are using a workspace instead of just a project (such as if you are using Cocoapods), go back 2 steps to the original folder that Xcode loaded and repeat the above 3 steps except starting by right clicking on the .xcworkspace file instead of the .xcodeproj file.
- Load your project/workspace again in Xcode, and the schemes should be rebuilt.
By the way, while we are at it, you do have the xcuserdata folder as an entry in your Git ignore file, right?
Hope you are all having a safe and happy holiday season. See you in 2024.
December 25, 2023, 1:09 pm
Well I just finished up the 2023 Advent of Code, and I must say that I must be getting senile or incompetent, because I found the problems to be challenging well above my pay grade.
However, it was fun to try and solve these problems using Swift.
Have a great holiday, everyone.
EDIT: Oh yeah, almost forgot, here is the link to my Github repo for this:
Advent of Code Solution Machine
November 16, 2023, 12:56 pm
Did you ever find yourself in a situation where you have a SQL Server database that has grown out of control, and you need to figure out where a call to insert a record into a particular table might be lurking in one of those triggers?
Oh sure, you can go into each table, expand the triggers folder in each table, then crack open each trigger showing and see if what you are looking for is there. For a database of non-trivial size, I would rather look up “tedium” in the dictionary.
Alas, there is a way to quickly screen down the number of places you may have to look for to find what you need. Open up your database and paste this text into the query window, of course replacing SearchText with whatever table name you need to search for:
SELECT so.name, text FROM sysobjects so, syscomments sc
WHERE TYPE = 'TR' AND so.id = sc.id AND text LIKE '%SearchText%' |
SELECT so.name, text FROM sysobjects so, syscomments sc
WHERE type = 'TR' AND so.id = sc.id AND text LIKE '%SearchText%'
For example, if you wanted to find any trigger that inserts a record into a table called Customers, you would do this query:
SELECT so.name, text FROM sysobjects so, syscomments sc
WHERE TYPE = 'TR' AND so.id = sc.id AND text LIKE '%insert into Customers%' |
SELECT so.name, text FROM sysobjects so, syscomments sc
WHERE type = 'TR' AND so.id = sc.id AND text LIKE '%insert into Customers%'
Keep in mind that unless you played with the settings or collation of your database, the case of the text in the LIKE statement above should not matter.
June 27, 2023, 8:56 pm
I am saving this here just in case the original source of this goes off line.
To download the drivers for Raspbian Buster, do these steps:
sudo rm -rf LCD-show
git clone https://github.com/goodtft/LCD-show.git
chmod -R 755 LCD-show
cd LCD-show/
sudo ./MHS35-show
To rotate the screen, do these steps:
cd LCD-show/
sudo ./rotate.sh 90
EDIT (10/4/2023):
When you set up the Raspberry Pi to use the LCD screen driver, it does disable the onboard HDMI output ports, so if you attach a cable there you will not get any video or audio.
If you want to reset your Raspberry Pi back to using the HDMI output then instead of the attached screen, do these steps:
cd LCD-show/
sudo ./LCD-hdmi
August 21, 2022, 9:59 pm
So once again I forgot to post about finishing up the Advent of Code. Here is my Swift code for the solutions:
Advent of Code Solution Machine
October 26, 2021, 2:45 pm
Due to a need to support older versions of .NET code and applications, you may find yourself staring at the need to call an async function from a standard synchronous function, and you don’t want to (or have the time for more likely) to go back and retrofit all of the calling functions with async/await functionality. Well brother, do I have a deal for you.
Here is how you can call the SendGrid SendEmailAsync function from within a regular C# function, and still be able to inspect the result of the task that runs the async method:
public bool SendPlainTextEmail(string subject, string body, List<string> toAddresses)
{
try
{
var apiKey = "Nice try Chachi, go ahead and insert your own SendGrid API key here";
var client = new SendGridClient(apiKey);
var from = new EmailAddress("your_verified_sender_email_address@sbemail.com", "Your verified SendGrid email sender name here");
var to = toAddresses.Select(x => new EmailAddress(x)).ToList();
var msg = MailHelper.CreateSingleEmailToMultipleRecipients(from, to, subject, body, string.Empty);
// Call the async function here and just wait for the results, no async function decorations required
var task = Task.Run(async () => await client.SendEmailAsync(msg).ConfigureAwait(false));
var result = task.Result.IsSuccessStatusCode;
return result;
}
catch (Exception ex)
{
throw ex;
}
} |
public bool SendPlainTextEmail(string subject, string body, List<string> toAddresses)
{
try
{
var apiKey = "Nice try Chachi, go ahead and insert your own SendGrid API key here";
var client = new SendGridClient(apiKey);
var from = new EmailAddress("your_verified_sender_email_address@sbemail.com", "Your verified SendGrid email sender name here");
var to = toAddresses.Select(x => new EmailAddress(x)).ToList();
var msg = MailHelper.CreateSingleEmailToMultipleRecipients(from, to, subject, body, string.Empty);
// Call the async function here and just wait for the results, no async function decorations required
var task = Task.Run(async () => await client.SendEmailAsync(msg).ConfigureAwait(false));
var result = task.Result.IsSuccessStatusCode;
return result;
}
catch (Exception ex)
{
throw ex;
}
}
I leave it up to you, the reader, to adapt this code for your own purposes, nefarious or otherwise. Regardless, the important stuff is happening with these two lines, which I have boiled down from above to remove the SendGrid cruft:
var task = Task.Run(async () => await theAsyncMethod());
var result = task.Result;
// do something here with the result |
var task = Task.Run(async () => await theAsyncMethod());
var result = task.Result;
// do something here with the result
In the interest of full disclosure, keep in mind you may need to do this import at or near the top of your source code file to be able to use the Task object in .NET:
using System.Threading.Tasks; |
using System.Threading.Tasks;
BTW, Happy Birthday to Seth MacFarlane, I can’t wait for season 3 of The Orville, March of 2022 can’t come soon enough.
September 29, 2021, 9:07 am
So you are humming along on a Swift project, and making excellent progress. However, somewhere in the back of your mind, you know that you should be doing things better, mainly due to your lack of experience in writing Swift code. In this case, you should be installing SwiftLint to help you with your code base.
The installation seems harmless enough:
brew install swiftlint
Once that finishes, however, you try to run a swiftlint command in the Terminal and are faced with this:
Fatal error: Loading sourcekitd.framework/Versions/A/sourcekitd failed: file SourceKittenFramework/library_wrapper.swift, line 39
Illegal instruction: 4
Fear not brave adventurer, you just have to get your Xcode select path correctly configured. With Xcode installed in the standard place, this command in the Terminal should get you all fixed up:
xcode-select -s /Applications/Xcode.app/Contents/Developer/
BTW, Happy Inventors’ Day to all my readers in Argentina.
February 7, 2021, 9:52 am
I can’t believe that I forgot to post about the 2020 Advent of Code until just now! Man I suck, but in truth, I have been a bit busy with my new gig.
There didn’t seem to be a puzzle this year that they kept building on like in years past. I actually kind of liked those kinds of challenges, but I will say that the 2020 set of challenges were fun to work on.
Please check out my Advent of Code Github repository:
https://github.com/Wave39/AdventOfCode
June 12, 2020, 2:14 pm
Some people wait a very long time, if ever, to do their Windows Updates. (For better or worse. Seriously people, run your Windows Updates.)
But this is a bit ridiculous…
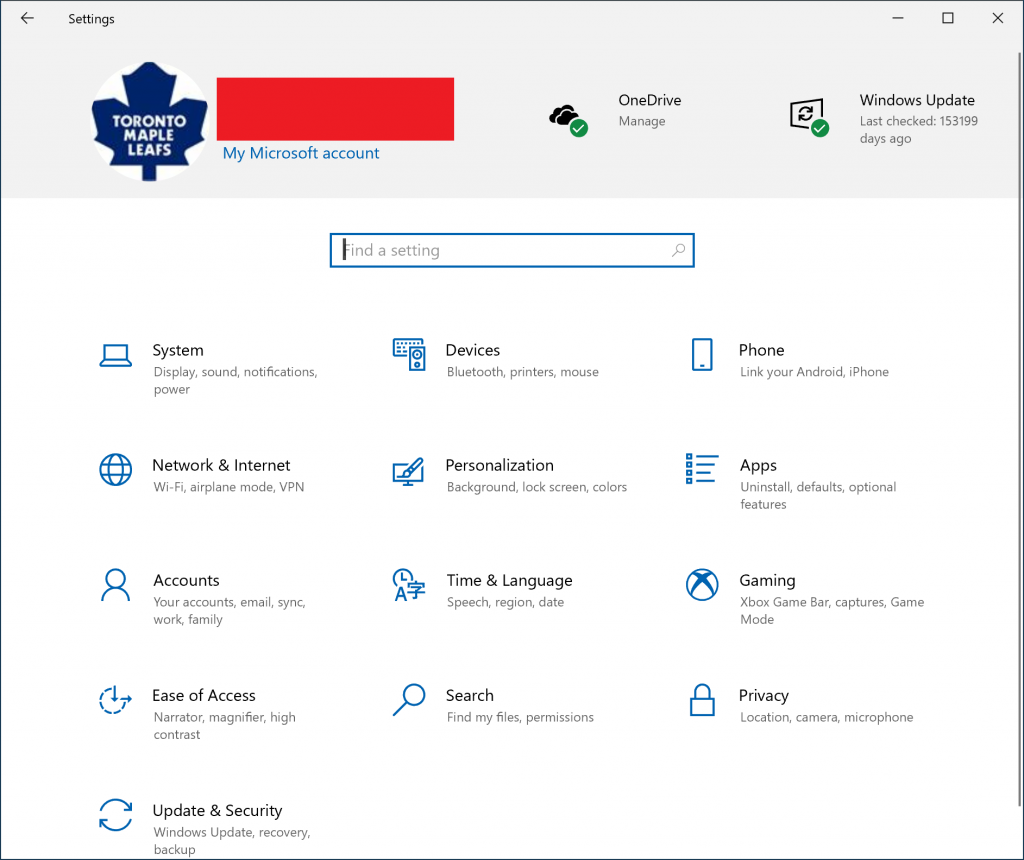
BTW, Happy Birthday to the National Baseball Hall of Fame, opened this day in 1939 in Cooperstown, New York. I sure wish we had baseball right about now…